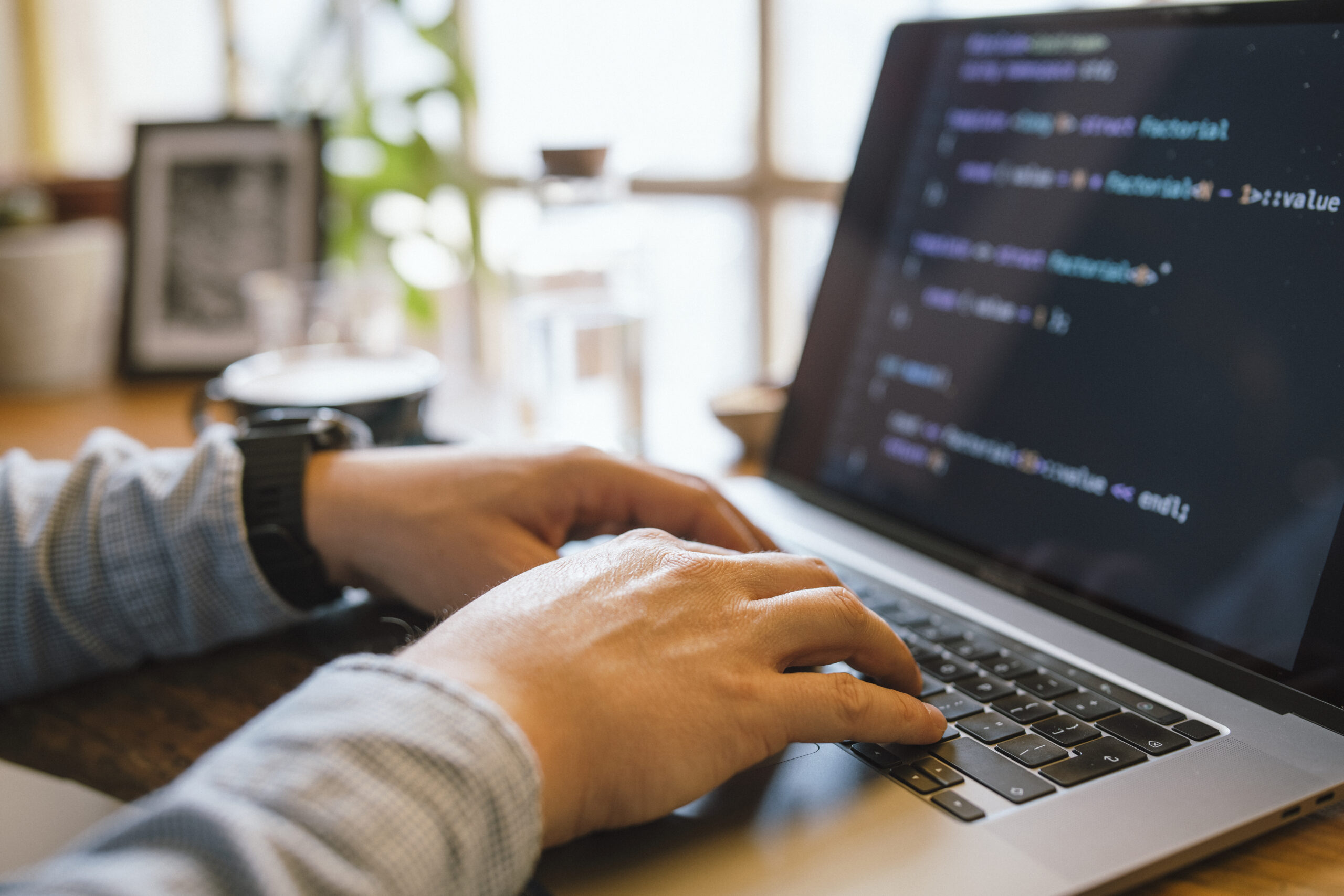
Debugging is Among the most important — nevertheless normally missed — abilities inside a developer’s toolkit. It is not almost repairing damaged code; it’s about comprehending how and why items go Improper, and Finding out to Assume methodically to resolve challenges efficiently. No matter whether you're a novice or even a seasoned developer, sharpening your debugging capabilities can preserve several hours of irritation and radically help your efficiency. Here's various strategies that will help builders stage up their debugging recreation by me, Gustavo Woltmann.
Master Your Tools
One of many quickest ways builders can elevate their debugging techniques is by mastering the equipment they use daily. Whilst composing code is a single A part of enhancement, figuring out the way to interact with it efficiently throughout execution is Similarly critical. Modern day development environments appear Geared up with effective debugging capabilities — but quite a few developers only scratch the area of what these equipment can perform.
Choose, one example is, an Integrated Enhancement Setting (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment help you set breakpoints, inspect the worth of variables at runtime, stage as a result of code line by line, and in many cases modify code within the fly. When used effectively, they Allow you to notice specifically how your code behaves in the course of execution, that is invaluable for monitoring down elusive bugs.
Browser developer resources, such as Chrome DevTools, are indispensable for entrance-stop developers. They help you inspect the DOM, watch network requests, watch genuine-time general performance metrics, and debug JavaScript within the browser. Mastering the console, resources, and community tabs can transform annoying UI issues into manageable responsibilities.
For backend or program-amount developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB offer deep Handle around operating processes and memory administration. Understanding these instruments can have a steeper Studying curve but pays off when debugging functionality challenges, memory leaks, or segmentation faults.
Beyond your IDE or debugger, come to be comfortable with Edition Handle devices like Git to be familiar with code history, obtain the exact moment bugs had been launched, and isolate problematic alterations.
In the long run, mastering your applications implies heading over and above default options and shortcuts — it’s about producing an personal familiarity with your progress atmosphere in order that when troubles occur, you’re not dropped in the dead of night. The higher you recognize your tools, the greater time you could expend resolving the particular challenge rather then fumbling via the process.
Reproduce the Problem
One of the most critical — and infrequently missed — techniques in effective debugging is reproducing the problem. Right before leaping to the code or producing guesses, developers have to have to create a dependable natural environment or circumstance in which the bug reliably appears. Without the need of reproducibility, repairing a bug will become a match of likelihood, often bringing about wasted time and fragile code modifications.
The first step in reproducing an issue is accumulating as much context as you possibly can. Talk to inquiries like: What actions triggered The difficulty? Which surroundings was it in — improvement, staging, or output? Are there any logs, screenshots, or error messages? The greater depth you have, the easier it will become to isolate the exact ailments below which the bug takes place.
After you’ve gathered plenty of details, try to recreate the challenge in your local setting. This may signify inputting the exact same information, simulating very similar user interactions, or mimicking process states. If the issue appears intermittently, take into consideration creating automated exams that replicate the sting cases or condition transitions included. These tests not merely assist expose the challenge but also avoid regressions Down the road.
Sometimes, The problem can be surroundings-precise — it might take place only on selected functioning methods, browsers, or beneath individual configurations. Applying tools like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating such bugs.
Reproducing the issue isn’t simply a move — it’s a state of mind. It needs persistence, observation, and also a methodical tactic. But as you can consistently recreate the bug, you happen to be by now midway to correcting it. Which has a reproducible state of affairs, you can use your debugging tools far more proficiently, exam opportunity fixes properly, and connect extra Evidently with your crew or consumers. It turns an abstract complaint into a concrete obstacle — Which’s wherever builders prosper.
Examine and Fully grasp the Mistake Messages
Error messages tend to be the most respected clues a developer has when some thing goes Incorrect. As opposed to viewing them as irritating interruptions, builders should really study to deal with error messages as immediate communications from the procedure. They generally inform you just what happened, where by it transpired, and from time to time even why it took place — if you understand how to interpret them.
Begin by reading the concept very carefully As well as in entire. Several developers, especially when underneath time strain, glance at the 1st line and right away start building assumptions. But further within the mistake stack or logs could lie the true root bring about. Don’t just copy and paste mistake messages into engines like google — read through and comprehend them initially.
Break the mistake down into components. Can it be a syntax error, a runtime exception, or simply a logic mistake? Does it position to a specific file and line variety? What module or function activated it? These questions can information your investigation and level you towards the responsible code.
It’s also valuable to understand the terminology on the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java generally comply with predictable styles, and Understanding to acknowledge these can drastically quicken your debugging course of action.
Some errors are obscure or generic, As well as in Individuals scenarios, it’s crucial to examine the context through which the mistake occurred. Check out similar log entries, input values, and up to date variations within the codebase.
Don’t forget about compiler or linter warnings possibly. These normally precede bigger troubles and supply hints about possible bugs.
Eventually, mistake messages are usually not your enemies—they’re your guides. Learning to interpret them appropriately turns chaos into clarity, supporting you pinpoint difficulties a lot quicker, minimize debugging time, and become a far more successful and self-assured developer.
Use Logging Sensibly
Logging is one of the most potent resources within a developer’s debugging toolkit. When made use of correctly, it offers serious-time insights into how an software behaves, encouraging you have an understanding of what’s happening under the hood without needing to pause execution or phase throughout the code line by line.
An excellent logging method begins with realizing what to log and at what degree. Typical logging ranges consist of DEBUG, Information, WARN, Mistake, and Lethal. Use DEBUG for specific diagnostic data for the duration of growth, Data for common functions (like profitable commence-ups), WARN for opportunity difficulties that don’t split the application, Mistake for genuine complications, and Lethal once the method can’t continue.
Stay clear of flooding your logs with abnormal or irrelevant knowledge. Excessive logging can obscure crucial messages and slow down your system. Center on essential occasions, point out alterations, input/output values, and important selection factors in your code.
Structure your log messages clearly and continuously. Incorporate context, such as timestamps, ask for IDs, and function names, so it’s simpler to trace troubles in distributed programs or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs Permit you to monitor how variables evolve, what disorders are satisfied, and what branches of logic are executed—all without halting This system. They’re Specifically important in creation environments where by stepping by means of code isn’t possible.
Moreover, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with monitoring dashboards.
Finally, sensible logging is about harmony and clarity. With a properly-assumed-out logging method, you may reduce the time it requires to spot concerns, get further visibility into your programs, and Enhance the Over-all maintainability and reliability of one's code.
Consider Similar to a Detective
Debugging is not only a complex endeavor—it's a type of investigation. To properly detect and fix bugs, developers need to approach the process like a detective fixing a thriller. This way of thinking allows break down intricate difficulties into workable components and stick to clues logically to uncover the basis lead to.
Start out by accumulating proof. Think about the indications of the problem: error messages, incorrect output, or functionality difficulties. The same as a detective surveys against the law scene, obtain just as much applicable information and facts as you could without leaping to conclusions. Use logs, exam instances, and user reports to piece together a transparent photograph of what’s going on.
Upcoming, sort hypotheses. Question by yourself: What could be producing this actions? Have any improvements not long ago been manufactured on the codebase? Has this situation transpired prior to under equivalent circumstances? The goal will be to slim down prospects and determine potential culprits.
Then, take a look at your theories systematically. Try and recreate the trouble in a controlled environment. When you suspect a particular function or ingredient, isolate it and confirm if the issue persists. Just like a detective conducting interviews, inquire your code questions and Permit the final results lead you nearer to the truth.
Pay back near attention to smaller specifics. Bugs often cover from the least envisioned areas—similar to a missing semicolon, an off-by-a person mistake, or perhaps a race affliction. Be comprehensive and affected individual, resisting the urge to patch the issue without having fully knowledge it. Temporary fixes may well hide the true problem, only for it to resurface afterwards.
Finally, preserve notes more info on Anything you attempted and figured out. Equally as detectives log their investigations, documenting your debugging method can help you save time for long term difficulties and help Other folks understand your reasoning.
By wondering like a detective, developers can sharpen their analytical competencies, method troubles methodically, and come to be more effective at uncovering hidden difficulties in complex techniques.
Write Exams
Composing checks is among the most effective approaches to transform your debugging skills and General growth effectiveness. Assessments not just aid catch bugs early and also function a security Web that offers you assurance when making modifications in your codebase. A properly-analyzed software is much easier to debug mainly because it allows you to pinpoint exactly exactly where and when an issue occurs.
Start with unit checks, which focus on personal features or modules. These tiny, isolated exams can rapidly reveal whether or not a specific piece of logic is Doing the job as envisioned. Any time a take a look at fails, you immediately know where by to glimpse, noticeably cutting down enough time put in debugging. Unit tests are especially practical for catching regression bugs—difficulties that reappear immediately after Formerly staying mounted.
Up coming, integrate integration checks and conclude-to-stop tests into your workflow. These assistance be sure that a variety of elements of your software work jointly easily. They’re especially practical for catching bugs that arise in sophisticated systems with many elements or services interacting. If a thing breaks, your exams can show you which Element of the pipeline failed and less than what problems.
Writing assessments also forces you to Assume critically about your code. To check a function thoroughly, you may need to understand its inputs, envisioned outputs, and edge circumstances. This volume of comprehension naturally qualified prospects to raised code construction and much less bugs.
When debugging a problem, producing a failing test that reproduces the bug might be a robust initial step. As soon as the check fails continuously, you are able to center on fixing the bug and observe your take a look at pass when the issue is solved. This solution ensures that precisely the same bug doesn’t return in the future.
In a nutshell, producing checks turns debugging from a aggravating guessing video game right into a structured and predictable procedure—aiding you capture extra bugs, faster and even more reliably.
Acquire Breaks
When debugging a tricky situation, it’s uncomplicated to be immersed in the problem—looking at your display for hrs, striving Option just after solution. But Probably the most underrated debugging equipment is just stepping absent. Having breaks allows you reset your intellect, reduce aggravation, and often see the issue from the new standpoint.
If you're much too near the code for much too extensive, cognitive exhaustion sets in. You might start overlooking obvious errors or misreading code that you wrote just several hours before. With this condition, your brain turns into much less effective at problem-resolving. A brief wander, a espresso split, or perhaps switching to a different task for ten–quarter-hour can refresh your target. Numerous builders report acquiring the basis of a difficulty after they've taken the perfect time to disconnect, letting their subconscious do the job from the track record.
Breaks also assist prevent burnout, In particular for the duration of for a longer time debugging sessions. Sitting down in front of a monitor, mentally caught, is not just unproductive but will also draining. Stepping absent lets you return with renewed Power and a clearer way of thinking. You could suddenly see a missing semicolon, a logic flaw, or a misplaced variable that eluded you in advance of.
Should you’re trapped, an excellent rule of thumb is usually to set a timer—debug actively for 45–60 minutes, then have a 5–ten moment split. Use that point to move all over, stretch, or do a thing unrelated to code. It may sense counterintuitive, Particularly underneath tight deadlines, but it surely in fact causes more quickly and more practical debugging In the end.
Briefly, taking breaks is just not an indication of weakness—it’s a wise system. It provides your Mind House to breathe, improves your viewpoint, and allows you stay away from the tunnel eyesight That always blocks your progress. Debugging can be a psychological puzzle, and relaxation is part of fixing it.
Study From Each Bug
Each and every bug you face is a lot more than just a temporary setback—It truly is a possibility to grow being a developer. Irrespective of whether it’s a syntax error, a logic flaw, or even a deep architectural situation, every one can instruct you one thing worthwhile when you take the time to reflect and evaluate what went Mistaken.
Start out by inquiring yourself a few essential issues as soon as the bug is fixed: What prompted it? Why did it go unnoticed? Could it have been caught earlier with much better methods like unit testing, code reviews, or logging? The answers often reveal blind places in the workflow or understanding and help you build more powerful coding routines shifting forward.
Documenting bugs can also be an excellent habit. Continue to keep a developer journal or manage a log in which you Notice down bugs you’ve encountered, how you solved them, and Everything you discovered. As time passes, you’ll begin to see styles—recurring troubles or widespread blunders—you can proactively keep away from.
In crew environments, sharing Everything you've learned from the bug with the peers may be Primarily highly effective. No matter whether it’s through a Slack information, a brief create-up, or A fast expertise-sharing session, aiding others steer clear of the similar situation boosts team performance and cultivates a more powerful learning lifestyle.
A lot more importantly, viewing bugs as classes shifts your frame of mind from aggravation to curiosity. In lieu of dreading bugs, you’ll start off appreciating them as important portions of your advancement journey. After all, several of the very best builders aren't those who compose fantastic code, but people who consistently understand from their issues.
Ultimately, Each individual bug you resolve provides a brand new layer on your talent set. So upcoming time you squash a bug, take a second to mirror—you’ll occur away a smarter, far more able developer due to it.
Summary
Enhancing your debugging techniques takes time, apply, and endurance — but the payoff is huge. It can make you a far more efficient, assured, and able developer. The subsequent time you might be knee-deep inside a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to be better at Everything you do.